포인터는 다른 변수의 위치를 가르키는 변수이다. 다음 그림과 같이 값을 담은 변수에 대하여 주소값을 가질 수 있으며 이는 프로그래밍에서 유용하게 활용 될 수 있다.
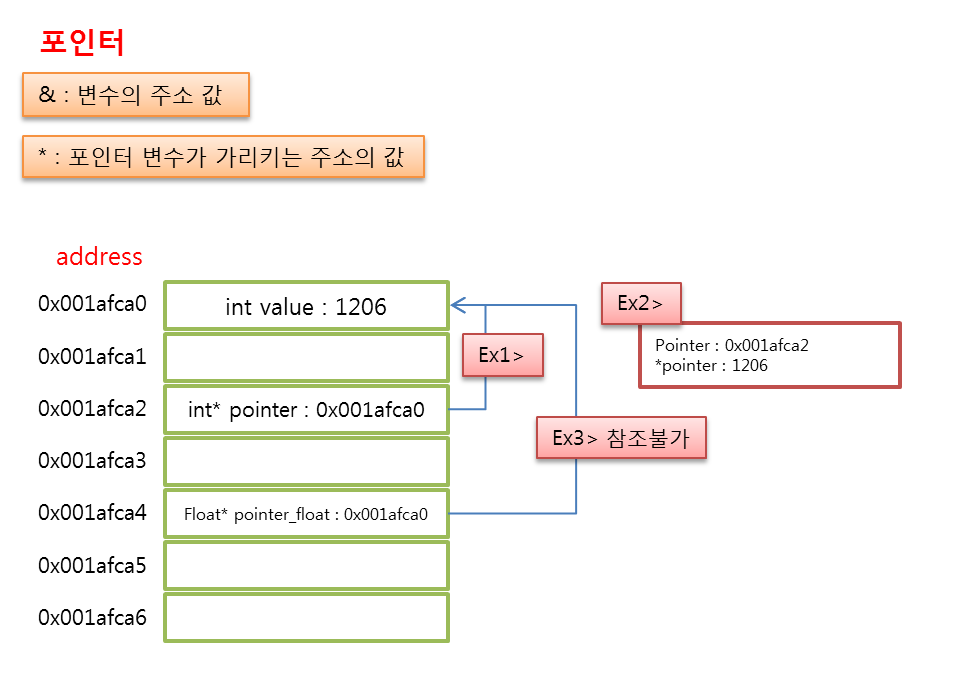
#include "stdafx.h"
#include <iostream>
using namespace std;
int _tmain(int argc, _TCHAR* argv[])
{
int value = 1206;
// ※ 포인터 변수는 항상 0 또는 NULL로 초기화 하고 사용할때는 항상 0 또는 NULL인지를 확인한다.
// - 0으로 초기화 하지 않는다면 쓰레기 값을 가지게 되고
// 만약 쓰레기 값이 다른 중요한 정보를 접근할 경우
// 심각한 문제가 발생할 여지가 있다.
int* pointer = NULL;
// Ex1> pointer는 포인터형 변수 이므로 value의 주소값만 받을 수 있다.
// value 앞에 &(Ampersand)를 붙여주면 주소값이 된다.
pointer = &value;
// [Error]
//pointer = value;
// Ex2> pointer가 가리키고 있는 값을 출력할때는 앞에 *를 붙여야 한다.
cout << "Ex2.\n";
cout << "ADDRESS : " << pointer << "\n";
cout << "VALUE : " << *pointer << "\n";
cout << "\n";
// Ex3> 포인터변수에 주소를 지정할때는 동일한 자료형의 주소만 받을 수 있다.
float* pointer_float;
float temp = (float)value;
pointer_float = &temp;
cout << "Ex3-1.\n";
cout << "VALUE : " << *pointer_float << "\n";
cout << "\n";
// [Error] : 다른 자료형의 포인트 변수라면 형변환을 해주면 에러가 나지는 않지만 쓰레기 값이 출력된다.
// - 이는 정수타입의 메모리를 실수타입으로 분석해버려서 이다.
float* pointer_temp;
pointer_temp = (float*)&value;
cout << "Ex3-2.\n";
cout << "VALUE : " << *pointer_temp << "\n";
cout << "\n";
// [Error] : 메모리에는 변수의 값만 저장하기 때문에 자료형을 알 수가 없기때문에 규칙위반이다.
//pointer_temp = &value;
// Ex4> 포인터변수에 동일한 자료형이 아닌 주소를 받아 유연하게 사용하려면 void*를 사용한다.
void* pointer_void;
pointer_void = &value;
// 사용할 때는 다시 형변환을 해주어야 한다.
int* pointer_test = (int*)pointer_void;
cout << "Ex4.\n";
cout << "ORG_ADDRESS : " << pointer_void << "\n";
cout << "NEW_ADDRESS : " << pointer_test << "\n";
// [Error] : 원래 자료형과 다른 포인터 형이므로 규칙위반이다.
// cout << "VALUE : " << *pointer_void << "\n";
cout << "VALUE : " << *pointer_test << "\n";
cout << "\n";
// Ex5> 포인터변수 크기는 시스템의 기본연산단위를 사용한다. 자료형별 차이는 없다.
// (16bit=>2byte, 32=>4byte, 64=>4byte)
cout << "Ex5.\n";
cout << "char* \t\t: " << sizeof(char*) << " bytes\n";
cout << "int* \t\t: " << sizeof(int*) << " bytes\n";
cout << "float* \t\t: " << sizeof(float*) << " bytes\n";
cout << "double* \t: " << sizeof(double*) << " bytes\n";
cout << "\n";
return 0;
}